MIT6.S081 Lab10
Lab10 Mmap
In this lab, we need to develop a weak
mmap(void* addr, size_t length, int prot, int flags, int fd, off_t offset)
, which have no designated addr
and prot
and flags
are also limited to READ, WRITE and both
and SHARED and PRIVATE
.
Also, we have to implement munmap(void *addr, size_t length)
, which won’t punch a hole in the middle of a region.
To make mmap fast return, we won’t allocate any physical pages for a mmap call, and instead, we just put the call into a vma
slot contains its meta information.
1 | struct vma{ |
In the lab website, lecturer has given us detailed information about how to write the code. And I want to introduce my design method which may be helpful to understand how to actually implement mmap.
I will throw some questions to help (you or me later) to understand why I do this
- Where to put
vma
in the memory?
1 | At first I want to let vma increase with proc->sz, which means each time a mmap is called we need to reserve some `complete 4k size` in user memory to be physically allcoated. |
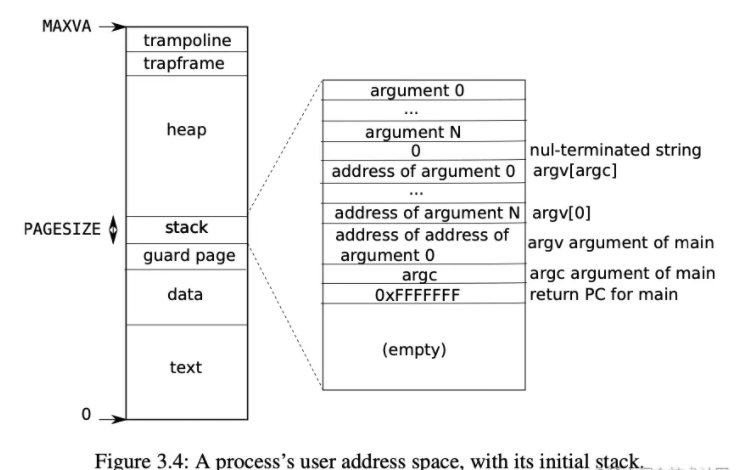
- When to allocate the physical page
1 | In the mmap() call, we just select an empty vma slot and put this information into the slot then return. |
1 | int do_mmap(struct vma* mmap, uint64 va){ |
- When allocate a physical page, we need to implement our own
uvmalloc
function to set the permission flags. - How to deal with munmap?
- In this lab, we just need to deal with 3 situation:
- free from beginning to some point before end
- free from some point after start to the end
- free all
- What should we do while unmaping?
- free whole 4k page if the entire 4k range is unmaped.
- If the 4k is being freed, we have to write back the content if
flag
set asMAP_SHARED
- If the beginning is free, we have to redirect
vma->offset
, so when read from file, the truefile offset
should beva - vma->vstart + vma->offset
- In this lab, we just need to deal with 3 situation:
1 | sys_munmap(){ |
- To deal with fork, although we copy vma from old to new, new pagetable won’t have to the corresponding pte on it. Because it just copy the pte range from [0, p->sz].
- Don’t forget to wipe out valid when proc is freed.