399. Evaluate Division
Description
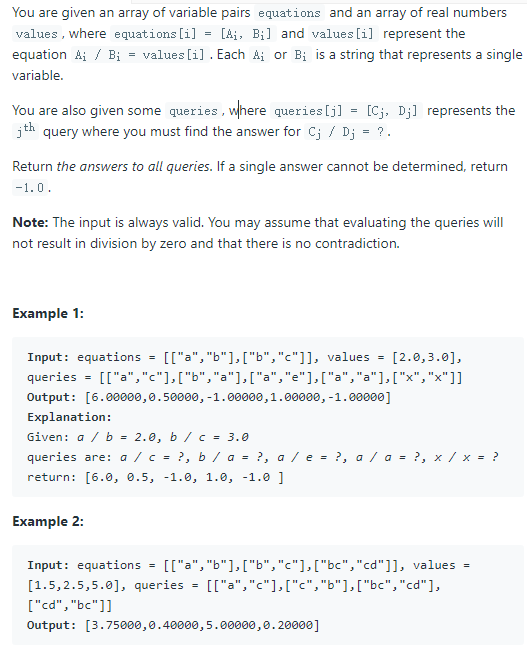
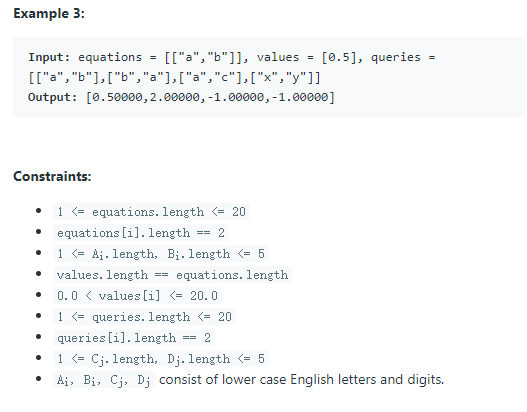
Solution
Record each equations’ string name and its corresponding index, used later.
Then do a floyd search
to all strings, find their division.
Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| class Solution { public: vector<double> calcEquation(vector<vector<string>>& equations, vector<double>& values, vector<vector<string>>& queries) { vector<string> names; unordered_map<string, unordered_map<string, double>> Map; for(int i = 0; i < equations.size(); i++){ if(Map.find(equations[i][0]) == Map.end()){ Map[equations[i][0]][equations[i][0]] = 1.0; names.push_back(equations[i][0]); } if(Map.find(equations[i][1]) == Map.end()){ Map[equations[i][1]][equations[i][1]] = 1.0; names.push_back(equations[i][1]); } Map[equations[i][0]][equations[i][1]] = values[i]; Map[equations[i][1]][equations[i][0]] = 1.0/values[i]; } for(int k = 0;k < Map.size();k++){ for(int i = 0; i < Map.size();i++){ for(int j = 0; j < Map.size(); j++){ if(i == j || i == k || j == k) continue; string a = names[i], b= names[j], c= names[k]; if(Map[a].find(c) != Map[a].end() && Map[b].find(c) != Map[b].end()){ Map[a][b] = Map[a][c] / Map[b][c]; Map[b][a] = Map[b][c] / Map[a][c]; } } } } vector<double> res; for(int i = 0; i < queries.size(); i++){ string a = queries[i][0], b = queries[i][1]; if(Map.find(a) == Map.end() || Map.find(b) == Map.end()) res.push_back(-1); else if(Map[a].find(b) == Map[a].end()) res.push_back(-1); else res.push_back(Map[a][b]); } return res; } };
|